HTTP Methods for Endpoints
API endpoints act as the access points through which clients (like applications or systems) can communicate with the API to carry out different actions. Each endpoint is usually linked to a specific HTTP method, such as GET, POST PUT, PATCH, or DELETE, which specifies the type of operation to be performed on the resource.
Each of you has a unique way of processing information. For this reason, we've structured our examples into both visual and written formats. If you value thorough explanations, proceed with the written guide below, which includes visual aids. However, if you enjoy learning through audio-visual content, check out the video tutorial on our YouTube channel. And, of course, you can combine reading and watching to fully grasp the process of utilizing different HTTP methods.
Prerequisites
We will use the Remote Agent in our example. Create and activate an agent. Learn more about Remote Agents here.
Create two new Data Schemas
:
Create a new Endpoint.
Save the Endpoint
.
Let's break down how different HTTP methods can be used using a library as an example.
Create a database
For further work with the library, we need to create a database, which we will do in the first task.
Configuration
Add a Task step:
- Connector: Microsoft SQL Database
- Agent: Remote Agent
- Output schema: Book
-
Configuration:
- fill the MSSQL Connection string and save it
- fill the MSSQL Statement and save it
IF OBJECT_ID('Library', 'U') IS NOT NULL
DROP TABLE Library;
-- if the table does not exist, create it
IF NOT EXISTS (SELECT * FROM sysobjects WHERE name='Library' AND xtype='U')
BEGIN
CREATE TABLE Library (
ID INT,
BookName VARCHAR(255),
Author VARCHAR(255)
);
END
-- inserting a static line
INSERT INTO Library (ID, BookName, Author)
VALUES (222, 'The Midnight Watch', 'David Dyer');
SELECT * FROM Library;
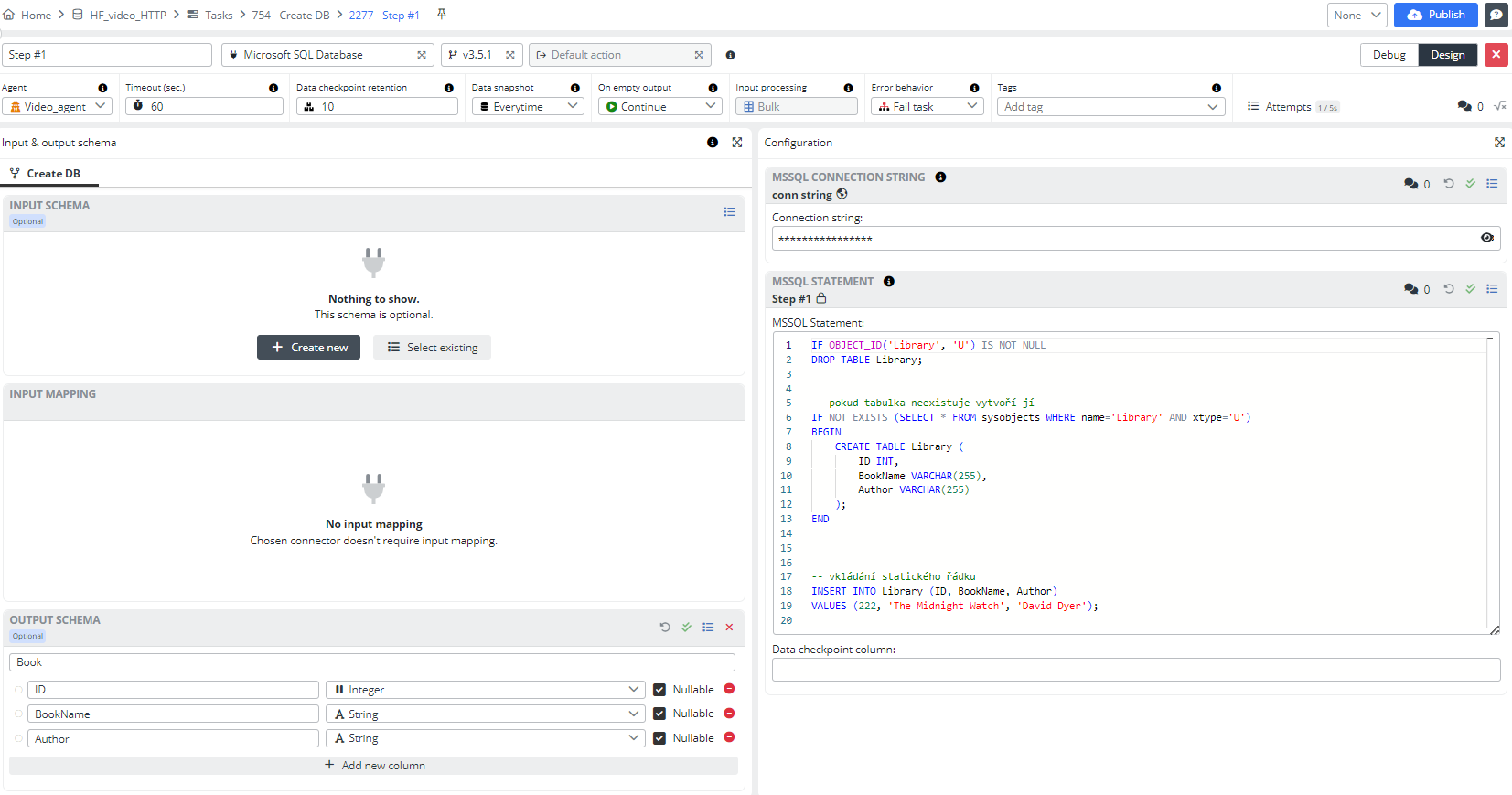
Press the Publish button. Start the task and go to the History.
Result - Creating a new table "Library"
In this task, we get a new table with data about the book, which we will work on within the following tasks.
POST - Add book
POST will send the book schema and return the entire library. We'll add one more book to the library.
Configuration
Add the name of the Task: POST Add book
Add a First Task step:
- Add the name of the Step: JS
- Connector: JS Mapper
- Agent: Background Agent
- Input schema: Book
- Output schema: Book
- Configuration:
return [
{
"ID": inputData[0].ID,
"BookName": inputData[0].BookName,
"Author": inputData[0].Author
}
];
Add a second Task step:
- Add the name of the Step: DB
- Connector: Microsoft SQL Database
- Agent: Remote Agent
- Input schema: Book
- Output schema: Book
-
Configuration:
- fill the MSSQL Connection string and save it
- fill the MSSQL Statement and save it
INSERT INTO Library (ID, BookName, Author)
VALUES (${input[0].ID}, ${input[0].BookName}, ${input[0].Author});
SELECT * FROM Library;
Close the Step, change the Task trigger to Endpoint
, and bind Endpoint to the Task.
Open the Endpoint and add the Input:
- Input Schema: Book
Save the Endpoint, go to the task, and pin the mapping if necessary.
Then go to Endpoint and add the Output:
- Output Schema: Book
- Output Step: DB
Save the Endpoint, go to Task, and pin the mapping.
Now go to Endpoint and pin output mapping.
Press the Save and Close button. Go to the Task.
Press the Publish button. Start the task and click on the History.
Result - The book has been added
In this task, we added a book to the library.
GET All books
Get a list of all the books in your library.
Configuration
Add the name of the Task: GET All books
Add a Task step:
- Add the name of the Step: DB
- Connector: Microsoft SQL Database
- Agent: Remote Agent
- Output schema: Book
-
Configuration:
- fill the MSSQL Connection string and save it
- fill the MSSQL Statement and save it
SELECT * FROM Library;
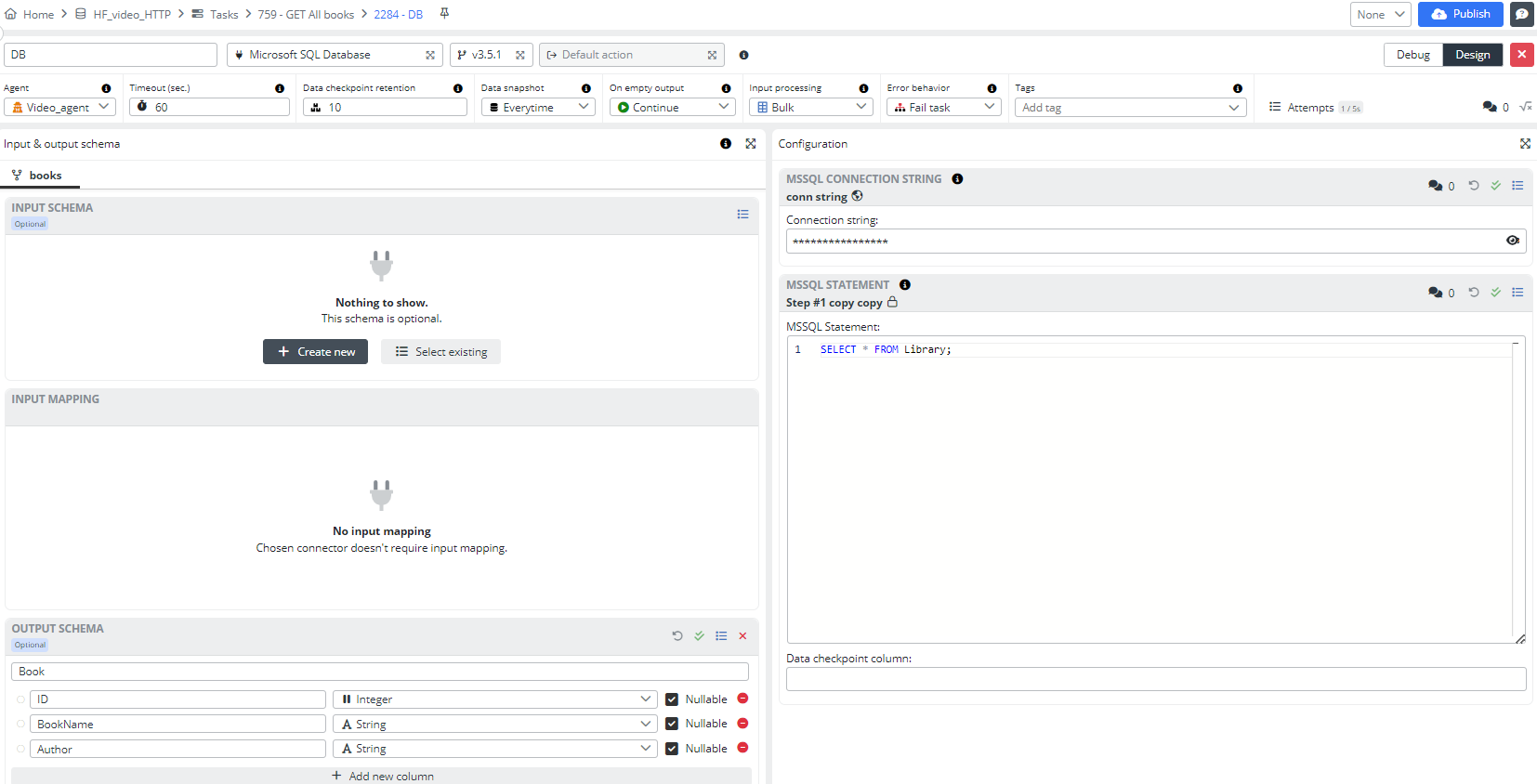
Close the Step, change the Task trigger to Endpoint
, and bind Endpoint to the Task.
Open the Endpoint and add the Output:
- Output Schema: Book
- Output Step: DB
Pin output mapping.
Press the Save and Close button. Go to the Task.
Press the Publish button. Start the task and click on the History.
Result - Creating a new table "Library"
In this task, we get a list of books from the library.
DELETE Book by ID
DELETE by ID - find the book, delete its record, and return the library without this record.
Configuration
Add the name of the Task: DELETE Book by ID Add a Task step:
- Add the name of the Step: DB
- Connector: Microsoft SQL Database
- Agent: Remote Agent
- Input schema: BookID
- Output schema: Book
-
Configuration:
- fill the MSSQL Connection string and save it
- fill the MSSQL Statement and save it
DELETE FROM Library WHERE ID = ${input[0].ID};
SELECT * FROM Library;
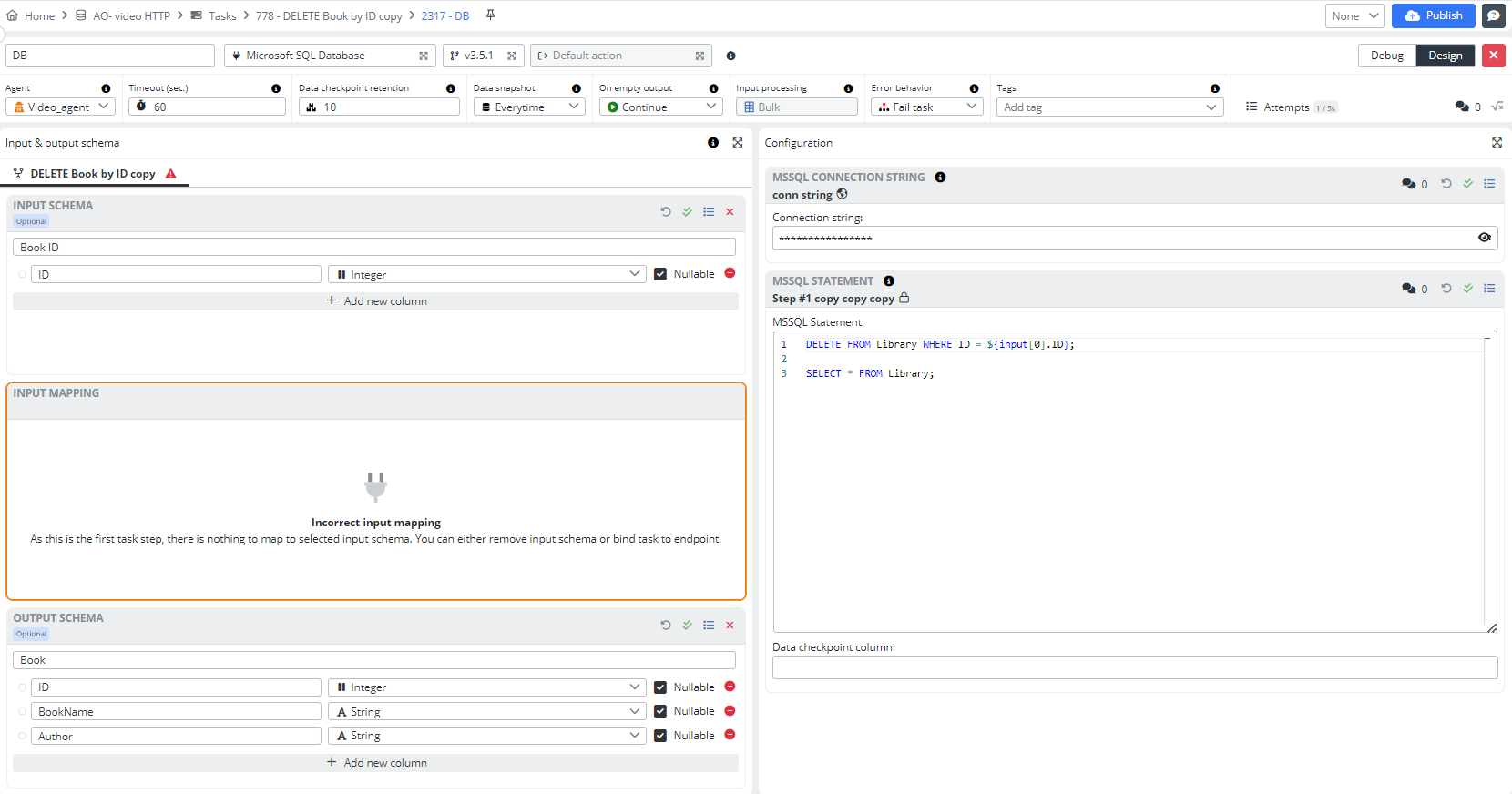
Close the Step, change the Task trigger to Endpoint
, and bind Endpoint to the Task.
Open the Endpoint and add the Input and Output:
- Input Schema: BookID
- Output Schema: Book
- Output Step: DB
Pin output mapping.
Press the Save and Close button. Go to the Task.
Press the Publish button. Start the task, and click on the History.
Result - Creating a new table "Library"
In this task, we get a list of books from the library.
PUT New Library
PUT discards the entire library and returns a whole new one.
Configuration
Add the name of the Task: PUT New Library
Add a First Task step:
- Add the name of the Step: JS
- Connector: JS Mapper
- Agent: Background Agent
- Input schema: Book
- Output schema: Book
- Configuration:
return [
{
"ID": inputData[0].ID,
"BookName": inputData[0].BookName,
"Author": inputData[0].Author
}
];
Add a second Task step:
- Add the name of the Step: DB
- Connector: Microsoft SQL Database
- Agent: Remote Agent
- Input schema: Book
- Output schema: Book
-
Configuration:
- fill the MSSQL Connection string and save it
- fill the MSSQL Statement and save it
IF OBJECT_ID('Library', 'U') IS NOT NULL
DROP TABLE Library;
-- if the table does not exist, create it
IF NOT EXISTS (SELECT * FROM sysobjects WHERE name='Library' AND xtype='U')
BEGIN
CREATE TABLE Library (
ID INT,
BookName VARCHAR(255),
Author VARCHAR(255)
);
END
INSERT INTO Library (ID, BookName, Author)
VALUES (${input[0].ID}, ${input[0].BookName}, ${input[0].Author});
INSERT INTO Library (ID, BookName, Author)
VALUES (${input[1].ID}, ${input[1].BookName}, ${input[1].Author});
INSERT INTO Library (ID, BookName, Author)
VALUES (${input[2].ID}, ${input[2].BookName}, ${input[2].Author});
SELECT * FROM Library;
Close the Step, change the Task trigger to Endpoint
, and bind Endpoint to the Task.
Open the Endpoint and add the Input:
- Input Schema: Book
Save the Endpoint, and add the Output:
- Output Schema: Book
- Output Step: DB
Press the Save and Close button. Go to the Task.
Press the Publish button. Start the task and click on the History.
Result - The book ID was edited
In this task, we edited a book ID in the library.
PATCH Book edit by ID
PATCH finds the book by ID, deletes its record, and replaces it with a new book.
Configuration
Add the name of the Task: PATCH Book edit by ID
Add a First Task step:
- Add the name of the Step: JS
- Connector: JS Mapper
- Agent: Background Agent
- Input schema: Book
- Output schema: Book
- Configuration:
return [
{
"ID": inputData[0].ID,
"BookName": inputData[0].BookName,
"Author": inputData[0].Author
}
];
Add a second Task step:
- Add the name of the Step: DB
- Connector: Microsoft SQL Database
- Agent: Remote Agent
- Input schema: Book
- Output schema: Book
-
Configuration:
- fill the MSSQL Connection string and save it
- fill the MSSQL Statement and save it
DELETE FROM Library WHERE ID = ${input[0].ID};
INSERT INTO Library (ID, BookName, Author)
VALUES (${input[0].ID}, ${input[0].BookName}, ${input[0].Author});
SELECT * FROM Library;
Close the Step, change the Task trigger to Endpoint
, and bind Endpoint to the Task.
Open the Endpoint and add the Input:
- Input Schema: Book
Save the Endpoint, and add the Output:
- Output Schema: Book
- Output Step: DB
Press the Save and Close button. Go to the Task.
Press the Publish button. Start the task and click on the History.
Result - The book ID was edited
In this task, we edited a book ID in the library.
We hope that you have successfully completed the task following our detailed instructions. Thank you for your attention, and we invite you to use our examples in the future.